Biga gamejam: Learning Unity, C#, Blender, and dusting off FL Studio
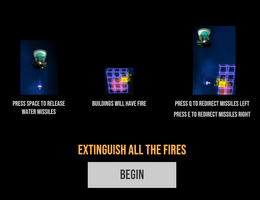
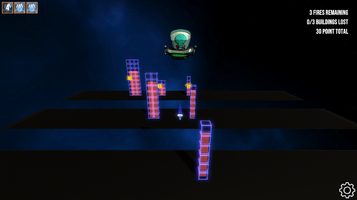
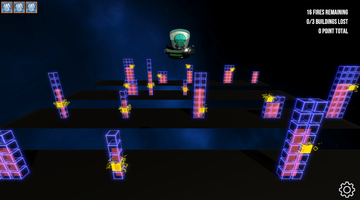
Intro
Decided to join a short gamejam to get back into creativity a bit. Haven't used Unity much or C# for that matter-- usually code in node + react for work. I've reached another point where my energy has been drained from hitting deadlines and writing mundane code + ui work for an eternity + 1 and am taking an extended break to try and recharge as of about a week and a half ago.
Gamejam was hosted by https://twitter.com/bigaarcade
Out the Gates + Personal Challenge
The gamejam lasted 5 days and as a personal challenge to myself I decided to not use any external assets or pre-built templates.
My reasoning for this is to challenge myself to learn as much as possible in as little time as possible. I've joined a handful of gamejams in the past (3-5 in the past 2 or 3 years about) and am always left working alone as the team usually ends up either prioritizing other things or giving excuses on why they haven't helped as much as they said they would.
This time I decided to do everything solo... the art, the music, the programming, game design, everything. Not as a flex, but more because the pattern that gets created as a result of me working with others is: if you tell me you're going to do something, and you end up not even trying to... I get discouraged pretty hard and it affects me keeping on with whatever it is I'm doing.
My motivation is amplified by others, but it can also be flattened entirely by them. It's something I've been working on for years but intrinsically it is a massive mountain to overcome. I believe this to stem from the sports I used to play but that's definitely a side topic.
screengrab of a concept art after about an hour:
Learning Blender
I've always loved character animation and design, I'm nowhere near half the talent I see online but am glad to have gone through a small learning phase these past 5 days.
I started trying to turn a 2D asset into a 3D model, a pre-requisite of the jam was to use 1 external asset from https://www.gamedeveloperstudio.com/. I found a pretty cool looking Alien character that was piloting a small 1-man spaceship. I think I can pretty much sum up the techniques I used into... start with a cube, cut it in half with a loop cut, delete the faces from the middle of the cube's X axis across the origin, apply a mirror modifier & begin modelling. After that is done the majority of what I found to work as a pattern was:
Select face, Extrude, Scale on all or on a specific axis, move edges or vertices around. Rinse and repeat.
When you're done add a subdivision modifier and apply it. Keep a copy of the non-applied model incase you need to upgrade it later.
Uv unwraps were a bit rough at first, but after having a proper go at the spaceship I was able to memorize a good bit of how to texture at a basic level at least.
Object mode -> U -> unwrap.
The only tricky part here was selecting edges with left-alt + left click edge in edge-select mode (something like a loop select) and then right clicking to create a seam. This basically lets you fix any areas where the texture map is too warped. Export the uvmap as a .png and send it to Photoshop for texturing. Bring it back to Blender then export the model with textures baked in, and uncheck the bottom checkboxes for size issues etc. Only caveat of exporting from Blender into Unity I ran into was making sure the origin, or pivot point, was dead center... and those two checkboxes im talking about in export options.
Here's the 3D Model I duct-taped together
C# : The ex girlfriend I needed during all those holidays spent alone in WoW...
I was a core Java dev for a while, I always liked the idea of design patterns, class Inheritance and Abstract classes. I really liked messing around with building random things that would utilize these... admittedly so, I was never really good at implementing them.
In my defense, we don't really have the need of them in the web / Application development industry. Definitely notice them being highly beneficial in game programming though. Finite State Machines are one of the more popular ones I've seen. Anyway it was nice getting back to a programming language that uses true Inheritance + extendable abstractions.
I'm sure to be coding things "non-optimally", but one thing I've learned as an engineer is that nothing we build is "fully-optimal".
Everything can always be improved upon, and things highly depend on how often things change-- be it a new feature or an addition to an existing one.
The bread and butter of my game were my MonoSingleton and CoreSingleton classes. Again, I'm sure there are better ways to handle things, but for a first-run at game development these two classes can solve a lot of problems.
public abstract class MonoSingleton<t> : MonoBehaviour where T : MonoSingleton<t> { static T _instance; public static T INSTANCE { get { if (_instance == null) { GameObject go = new(typeof(T).Name); _instance = go.AddComponent<t>(); } return _instance; } } public void Init() { } }
Here's an early version of buildings with fires being extinguished via water missiles
I'm still not used to using a UI for any code-related things, if I can have it in code I will prefer that... it's something about not really knowing much about the Unity editor, combined with things randomly going missing trying to find some missing script or GameObject.
The above code looks to solve that by allowing a MonoBehaviour to be accessed as a Singleton via `SomeBehaviourName.INSTANCE.DoSomething();`
The `Init()` method's purpose is to have an initialization chain that I chose to use an IEnumerator to run a Coroutine off of. This way you could have a central point of entry into your game via code.
If you don't need a MonoBehaviour, and want an easy way to extend a traditional Singleton, here is the code:
public abstract class CoreSingleton where T : CoreSingleton, new() { private static readonly T _instance = new(); static CoreSingleton() { } public static T INSTANCE { get { return _instance; } } public void Init() { } }
If you're not familiar with generics (<T>), this is how you would extend either of the above classes:
public class HudController : MonoSingleton<HudController> {
Call `HudController.INSTANCE.Init()` from anywhere in your code and you're gtg.
Some trickier parts off the top of my head were grabbing reference to a GameObject that needed to start out as inactive. GameObject.Find does not return inactive objects from the ui. Solution to this is to pass a bool parameter as true into `FindObjectOfType` method.
i.e. FindObjectOfType<InactiveGameObjectName>(true).gameObject;
What I started doing was creating empty classes named "InactiveNameGo" that extended MonoBehaviour to put on the GameObject I needed to access (that had an inactive state or a parent with an inactive state).
Again, 100% sure there has to be a better way on this but until then this is what got me through this gamejam.
I managed to get a decent level generator script running that would build the buildings from the ground up using a Coroutine to simulate blocks stacking on each other... during this time a ridiculously tricky-to-figure out bug punched me in the stomache as it ended up being non-code related but a setting in Unity to fix... it stemmed from trying to move a CharacterController via transform.position = ... or .Translate instead of using the built-in .Move method from CharacterController class ... there is a setting in Edit > Project Settings > Physics > Auto Sync Transforms ... this checkbox solved the problem.
Here's what the level generator + the position bug looked like
My EUREKA moment...
I had planned to use the level generator to try to make a massive circular city ... just spawning along an arch infinitely sounded pretty cool. Didn't get that far, but when I made moving into the z-axis possible it definitely felt great.
Another thing this shows is I tried upgrading the art a bit, specifically learned how to use Particle emitters and use maps + Post Processing with a Bloom filter in order to get those glowy edges.
Topics I need to study on:
- Canvas -> this seems like a damn rabbit hole starting out, with so many options and differences between scaling to screen size, overlay, world location, alignments, panels... etc.
- Better way to access / initialize inactive GameObjects
- Scriptable Objects (didn't touch them this run, but they seem to be the Model in a MVC pattern)
- Scene transitions / loading ... one of the many issues I ran across was my Cinemachine camera would take a bit to move into position, since the level was being generated dynamically and I would have to re-attach the Camera if the player had to re-spawn... on the initial spawn the camera takes 2-3 seconds to move into position as the level is being generated... I would like to find a way to have that initial camera movement smoother... or transition into it from another camera possibly.
Joy
I had a lot of fun these past 5 days ngl... Seeing the work-in-progress of other team's / engineers was also pretty cool. I feel a lot of creativity has been siphoned near-completely out of my industry and seeing these ideas as they come to life in game development is definitely a decent charge of energy into my soul-bones. There are so many talented bastiages out here, I'm going to have fun playing any of the public submissions in the next few days.
I haven't recorded a song from the ground up in an eternity... it felt really good to connect a midi keyboard and dive into FL Studio. Luckily I didn't have to learn too much there, I've been messing around with DAWs since I was around 11 years old back when Cakewalk, Acid, and a few others were around. Coolest feeling was playing a looped video of the game with no audio and messing around on keyboard until I found something I felt went with it.
What I really like from the short time I've been on Unity
They did an amazing job with the engine tbh. I imagine all the editor options and extensions offer MASSIVE quality of life improvements and would make a lot of things much easier, given you have a proper team to run with.
It's dangerous to go alone.
Most low-key sage advice from a video game I've seen.
If you're reading this hope you're staying positive & having a good day. Too much negative in the world not to.
there's definitely a few bugs floating around but the concept of the game is there... just imagine being chased by a swarm of alien mini-ships... and the building fires generated by random falling meteors ( :
- mimic
Files
Aliro1
one being, alone, betrayed by the worlds he loves.
Status | Prototype |
Author | banzaiMimic |
Tags | 3D, Low-poly, Unreal Engine |
More posts
- Unity vs UE5 + dev log updateApr 02, 2024
- ECS Dots console bugs won't let me keep going with itSep 10, 2023
Leave a comment
Log in with itch.io to leave a comment.